Horizontal Bar Graphs with SGX-120L Displays
(SGX-120L has been discontinued for many years; reference only.)
Although the SGX-120L serial graphics LCD can easily plot graphs using the Line and Line-To instructions, a simple trick can be used to generate seamless bar graphs. This app note shows how, using a Parallax BASIC Stamp II.
Horizontal Bar Graphs on Graphics LCDs
With a graphics LCD, the simplest way to create a bar graph is to generate a line with length proportional to the desired quantity. However, by borrowing a trick from text LCDs (which can't draw lines), we can create bars of different widths and patterns.
The basic idea is to take the desired bar length and divide it by the maximum character width (6 pixels in this case). Fill the bar in with as many 6-pixel-wide blocks as possible. Then pad the end of the bar with one additional character, 1 to 5 pixels wide to take up the remainder. Use blank spaces to erase any leftover characters from previous graphs and you're done.
An advantage of using this technique on a true graphics LCD like the SGX-120L is that there are no blank spaces between character positions as there are on alphanumeric LCDs. With custom characters, you can even do patterns, like the shaded bar in the photo.
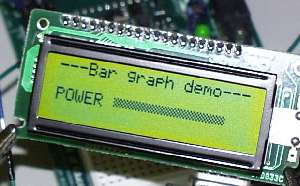
Since there are 6 pixels per character block, and the SGX-120L is 20 columns wide, we can depict values from 0 to 120 (assuming that the full screen width is used; here it isn't).
SGX-120L experts may be wondering why we're bothering with a custom font when the display's Byte-Write capability could also be used to draw bars. The reason is speed. Each font character fills a 6-byte space on the screen with only 1 byte sent serially. Byte-Write is an escape sequence that sends 3 bytes to fill a 1-byte space. So the custom-font technique is nearly 18x faster.
Configuring the SGX-120
Fonts used by the SGX-120 display are actually bitmaps stored in EEPROM. To add custom characters, use a Paint program to add the desired bitmaps to the font, download it to the display, and transfer it into EEPROM.
Here is the modified font we used to create the demo.
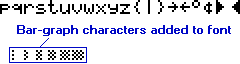
If you plan to run the demo program, download the modified font graphic. Use the downloading software that came with your SGX-120L, or visit our downloads page.
Hookup for Demo
The schematic at right shows how to connect the display to the
BS2 for the demo. Data for the graph comes from the pot/capacitor
hookup shown. If you want to omit the pot, you may, but you will
have to work out some other way to supply data to the bar-graph
routine.
Running the BS2 Program
The BS2 program is listed below. Comments explain most of the
mechanics of it. It makes generous use of a lesser-known features
of the BS2 Serout
instruction, the REP
(repeat) modifier.
Within a Serout
list (inside the square brackets
[...]), REP
causes a particular byte to be sent
repeatedly. For example,
serout pin,baudmode,[REP "X" \26]
..causes 26 Xs to be sent. A couple handy aspects of REP are that..
- The number of reps can be a variable.
- If the number of reps is 0, nothing is sent.
In our bar-graph application, we divide the value to be graphed by 6 (the max number of vertical bars in a single character position), and print that number of 6-bar character blocks. Then we take the remainder of division by 6 and print the appropriate bar block (1 to 5). The rest of the bar-graph width gets filled with blank spaces.
' Program: HGRAF2.BS2 (Horizontal bar graphs for BS2/SGX-120L) ' This program demonstrates how to generate horizontal bar ' graphs with the BASIC Stamp 2 controller and SGX-120L ' serial graphics LCD module (www.seetron.com). Before ' running this program, the SGX-120L must be loaded with the ' bar-graph symbols using the download utility included on ' the SGX-120L disk or on the Seetron downloads page, ' www.seetron.com/dl.shtml. The bitmap file to use is in ' www.seetron.com/charbg2.zip. Load the .BMP file to EEPROM ' screen 1 (second font screen). Set the SGX-120L for ' 9600 bps and connect its serial input to BS2 pin P0. ' Connect a 10k pot/0.1uF cap to pin P1 as shown in the ' schematic (or in Stamp manual under RCTIME). ' ==SGX-120L CONTROL CONSTANTS========================= N9600 con $4054 ' BS2 baudmode constant, 9600 bps. S_PIN con 0 ' Serout pin to SGX-120. CLRLCD con 12 ' ASCII form feed = clear screen. CTL_P con 16 ' Control-P (position cursor). ' ==BAR-GRAPH CONSTANTS================================ WIDTH con 14 ' Max width in characters of bar. MAXBAR con width * 6 ' Max counts per bar. FULBAR con 178 ' ASCII value of full (6-pixel) bar. BASBAR con 172 ' ASCII value of 0 bar (blank). ' ==PROGRAM VARIABLES================================== graf var word ' Value to be graphed. bars var byte ' Number of full (6-pixel) bars to draw. bal var byte ' Balance left after all 6-pix bars drawn. pad var byte ' Number of spaces to pad to fill width. balF var bit ' Is a 'bal' character needed? (1=yes, 0=no). ' ==DEMO PROGRAM======================================= pause 1000 ' Wait for LCD to initialize. serout S_PIN,N9600,[CLRLCD,"---Bar graph demo---",CTL_P,104,"POWER"] Again: gosub getPotVal ' Sample the pot. serout S_PIN,N9600,[CTL_P,110] ' Move to pos 46 (64+46 =110). gosub barGraph ' Display bar graph. goto Again ' Do it continuously. ' ==SUBROUTINES======================================== ' BARGRAPH: Take the value in 'graf' and present it as a ' horizontal bar graph from the current cursor location with a ' total width (in characters) set by the WIDTH constant. Each ' character position can represent a maximum value of 6 using ' the FULBAR character (a 6-pixel-wide block character added ' to the text font). The routine calculates how many full ' bars to use by dividing by 6. If there's a remainder after ' dividing by 6, the routine tacks on a partial-bar character ' (1 to 5 pixels wide) to represent the balance('bal'). It pads ' the remainder of the bar width with blank spaces in order to ' erase any leftovers from previous graphs. barGraph: bars = (graf max MAXBAR)/6 ' One full bar for each 6 graph units. bal = (graf max MAXBAR) // 6 ' Bal is remainder after division by 6. balF = bal max 1 ' If bal not = to 0 then balF=1. pad = WIDTH - (bars+balF) ' Number of spaces to fill bar width. serout S_PIN,N9600,[rep FULBAR \bars, rep (bal+BASBAR) \balF, rep " " \pad] return ' GETPOTVAL: For demo data, take a pot measurement through pin 1. Cap is ' 0.1uF to +5V; pot is 10k to ground, as shown in BS2 manual RCTIME entry. getPotVal: ' Output pot value high 1: pause 1 ' Discharge cap on pin 1. rctime 1,1,graf ' Measure charge time (approx. 0-600) graf = graf/6 ' Scale to 0-50 approx. return
Summary and Modifications
Modifying the bar-graph code is pretty straightforward. First, set the constant WIDTH at the beginning of the program to the maximum width (in characters) that you want the bar to cover. The maximum value that the bar can display will be 6x WIDTH.
Before a GOSUB barGraph
, make sure to position the
cursor to the desired starting position on the LCD screen. It's
probably obvious, but that position should be WIDTH or more
characters from the right-hand edge of the screen, or the bar will
wrap around.
Be sure that the SGX-120L has font-scaling turned off (the default), unless you have taken its effect into account in setting the WIDTH constant, etc.
Finally, put the value you want to graph into the variable
graf and GOSUB barGraph
. After the bar
graph has been generated, the cursor will be in the next character
position after the end of the graph.
The bar graph can be updated rapidly for a nice, animated effect. You may want to play with different graphical effects, including variations on the block characters used to make the bar, inverse-video (CTL-B) for white-on-black bars, etc.